How to create Scalable Applications for a Developer By Gustavo Woltmann
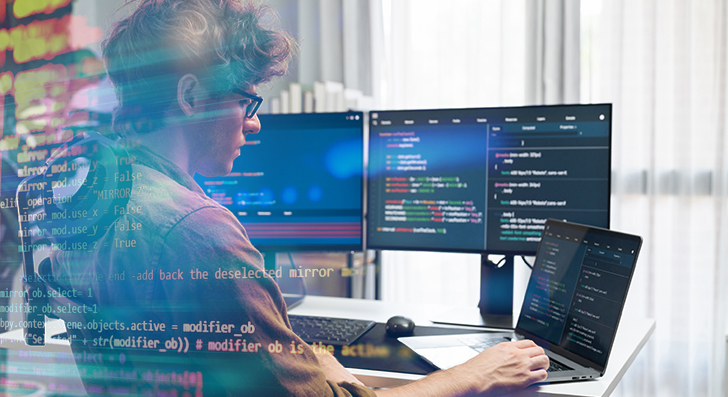
Scalability implies your software can take care of progress—much more users, additional knowledge, and even more visitors—without breaking. For a developer, constructing with scalability in mind saves time and worry later on. Here’s a transparent and useful guide to assist you to commence by Gustavo Woltmann.
Design for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs fail if they develop rapid simply because the first style can’t manage the additional load. Like a developer, you have to Assume early about how your technique will behave stressed.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases exactly where everything is tightly connected. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial sections. Each module or assistance can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it want to manage one million buyers or simply a hundred? Select the appropriate form—relational or NoSQL—based upon how your knowledge will improve. Approach for sharding, indexing, and backups early, even if you don’t require them but.
One more significant point is to prevent hardcoding assumptions. Don’t compose code that only is effective less than current circumstances. Take into consideration what would come about If the person foundation doubled tomorrow. Would your application crash? Would the database decelerate?
Use structure styles that aid scaling, like information queues or party-pushed units. These assistance your application cope with additional requests with out acquiring overloaded.
Whenever you Develop with scalability in mind, you are not just making ready for achievement—you are decreasing foreseeable future head aches. A effectively-planned system is less complicated to take care of, adapt, and increase. It’s greater to organize early than to rebuild later.
Use the appropriate Database
Choosing the right databases is actually a important part of setting up scalable apps. Not all databases are developed the identical, and using the wrong you can slow you down or even bring about failures as your app grows.
Start by knowledge your info. Can it be really structured, like rows in a very table? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They're strong with associations, transactions, and consistency. Additionally they assist scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted traffic and data.
When your data is much more adaptable—like consumer activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured facts and can scale horizontally far more easily.
Also, look at your study and publish styles. Have you been executing lots of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Investigate databases that can deal with significant generate throughput, or perhaps function-dependent details storage methods like Apache Kafka (for short term facts streams).
It’s also wise to Consider in advance. You might not have to have Sophisticated scaling functions now, but selecting a databases that supports them suggests you received’t have to have to modify later on.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And normally monitor databases performance as you expand.
In a nutshell, the correct database is determined by your app’s structure, speed needs, And exactly how you hope it to mature. Choose time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Speedy code is essential to scalability. As your application grows, each and every tiny delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Avoid repeating logic and take away nearly anything needless. Don’t choose the most elaborate Resolution if a simple a person performs. Keep your capabilities limited, focused, and easy to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or utilizes far too much memory.
Following, look at your databases queries. These typically gradual items down more than the code by itself. Make sure Every single query only asks for the information you truly need to have. Avoid Decide on *, which fetches every little thing, and in its place pick unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily across massive tables.
For those who recognize the exact same knowledge remaining requested many times, use caching. Shop the final results quickly utilizing equipment like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with a hundred documents may well crash if they click here have to take care of one million.
To put it briefly, scalable applications are fast apps. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to deal with far more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s where load balancing and caching come in. Both of these applications enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server carrying out each of the operate, the load balancer routes consumers to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this very easy to setup.
Caching is about storing information quickly so it could be reused swiftly. When users ask for the identical information once again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may provide it in the cache.
There's two frequent different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching reduces databases load, increases speed, and will make your app additional effective.
Use caching for things which don’t change typically. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are simple but strong applications. With each other, they assist your application handle a lot more buyers, stay rapidly, and Get better from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To construct scalable programs, you require tools that let your app increase quickly. That’s in which cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you hire servers and services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic boosts, you could increase extra means with just some clicks or automatically using auto-scaling. When traffic drops, you'll be able to scale down to save cash.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on building your application in lieu of taking care of infrastructure.
Containers are A different essential Instrument. A container packages your application and anything it ought to run—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent elements of your application into expert services. You'll be able to update or scale pieces independently, that's great for effectiveness and reliability.
To put it briefly, making use of cloud and container applications signifies it is possible to scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no limits, commence applying these resources early. They help save time, reduce chance, and help you remain centered on setting up, not repairing.
Monitor Every little thing
When you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place difficulties early, and make better choices as your app grows. It’s a essential Element of developing scalable techniques.
Start out by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app as well. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and the place they arise. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. Such as, If the reaction time goes above a limit or simply a company goes down, you'll want to get notified promptly. This can help you correct troubles quickly, frequently prior to buyers even see.
Checking is additionally valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the proper applications in position, you stay in control.
In a nutshell, checking aids you keep your app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your system and making certain it works properly, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps need to have a strong foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper applications, you'll be able to build apps that mature smoothly with no breaking stressed. Begin smaller, think huge, and Make smart.